Load Testing with Playwright for Java
In this tutorial, you'll learn how to reuse existing Playwright tests written in Java to quickly set up and run a browser-based load test using the automation as code approach.
Get Step SaaS for free to follow this tutorial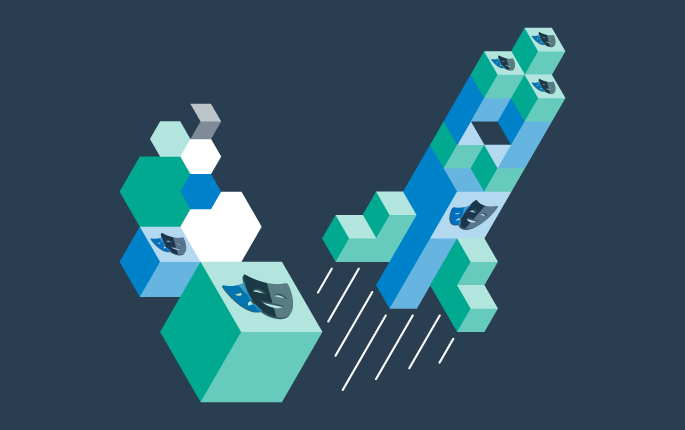
Prerequisites
- Access to a Step cluster: Get started quickly by setting up a SaaS cluster in the Step Portal, or, if preferred, follow the Installation page to configure your own on-premise cluster.
- Maven: Ensure that Apache Maven is installed on your local machine. You can download it from the official Maven website and follow the installation instructions provided here.
Test scenario
For the sake of this tutorial, we’ll simulate 10 parallel users visiting our demo online store. The user journey that we’ll simulate consists of the following actions: navigate to the online store, search for a product, add it to the shopping cart, and complete the order.
For this simulation we have an existing Playwright test written in Java: Playwright sample project
Checkout the sample
Clone the sample project from GitHub:
git clone https://github.com/exense/step-samples
Navigate to the sample directory:
cd step-samples/automation-packages/load-testing-playwright
Project structure
The project load-testing-playwright
is a standard maven project ready to build. It contains the following files:
Playwright test
The script that simulates our user journey using Playwright is contained in the class PlaywrightKeywords.java. The code is encapsulated within a method annotated with @Keyword
, which declares it as a Keyword (an atomic step that can be referenced in the load test plan).
For more details about Keywords, refer to the official documentation of the Keyword API.
Automation package with load test plan
The plan configuring the load test is defined in the descriptor of the automation package: src/main/resources/automation-package.yaml
. The example simulates 10 parallel users, each placing 10 orders in sequence. As you can see, the specification is straightforward – the complete definition is shown below.
---
schemaVersion: 1.0.0
name: "load-testing-playwright-automation-package"
plans:
- name: "OpenCart Playwright Load Test"
# Automatically determine and provision the agents (workers)
agents: auto_detect
root:
# Declare a thread group with 10 concurrent users
threadGroup:
users: 10
# Perform 100 iterations per user
iterations: 100
children:
- callKeyword:
# Each iteration executes the test defined as Keyword
keyword: "Buy MacBook in OpenCart"
For more details about the syntax, refer to the official documentation of the Automation Package Descriptor.
Maven Project
The file pom.xml
defines how the maven project and thus the automation package is built and how it is executed in Step.
The section relevant to the execution of the load test is shown here:
<build><plugins><plugin>
<groupId>ch.exense.step</groupId>
<artifactId>step-maven-plugin</artifactId>
<executions>
<execution>
<id>execute-automation-package</id>
<phase>integration-test</phase>
<configuration>
<url>${step.url}</url>
<authToken>${step.auth-token}</authToken>
<stepProjectName>${step.step-project-name}</stepProjectName>
<executionParameters>
<env>TEST</env>
</executionParameters>
<waitForExecution>true</waitForExecution>
<executionResultTimeoutS>600</executionResultTimeoutS>
<ensureExecutionSuccess>true</ensureExecutionSuccess>
</configuration>
<goals>
<goal>execute-automation-package</goal>
</goals>
</execution>
</executions>
</plugin></plugins></build>
These definitions ensure that during the integration-test
phase of maven (which is performed as a precondition of the mvn verify
command), the execute-automation-package
goal provided by the Step maven plugin is run. For more information about the various maven phases, see this reference.
You will need to provide the URL to your Step cluster, as well as the project name and API key (refer to Generate an API Key). In this example, the actual values are referencing variables which are conveniently defined at the beginning of the file. Make sure to adjust these values to your setup.
The other configuration parameters specify parameters to pass to Step for the actual execution (executionParameters
), tell maven to wait for the execution result for a maximum of 10 minutes (waitForExecution
and executionTimeoutS
), and finally, to check that the execution in Step was indeed successful (ensureExecutionSuccess
).
Refer to the official documentation of the Maven Plugin for more details.
Execute locally
During development or for debugging purposes, you can execute the load test locally using the following command:
mvn test -Dtest="RunAutomationPackageTest"
Execute in Step
To trigger the execution in a Step cluster, run the following maven command:
mvn verify -DskipTests
The load test is executed in Step according to the plan specified in resources/automation-package.yaml
. The maven project is configured to wait for the execution to complete.
The command outputs a direct link to the execution report in Step:
[INFO] Execution(s) started in Step:
[INFO] - 'OpenCart Playwright Load Test' (https://<Host name of your Step cluster>/#/executions/6719050949d80a76dc3edaa2)
[INFO] Waiting for execution(s) to complete...
[INFO] Execution 'OpenCart Playwright Load Test' (https://stepee-nhy.stepcloud-test.ch/#/executions/6719050949d80a76dc3edaa2) succeeded. Result status was PASSED
Analyze the result
Open the execution report in Step by clicking the direct link printed by the command above.
In the Performance tab, you can explore detailed performance metrics:

There you’ll find all performance metrics:
- The total execution time of our Keyword: “Buy MacBook in OpenCart”
- The series related to the performance measurements made within the Keyword
Review the transaction statistics
At the bottom of the Performance view, you’ll find an aggregation of performance metrics for the selected time frame:

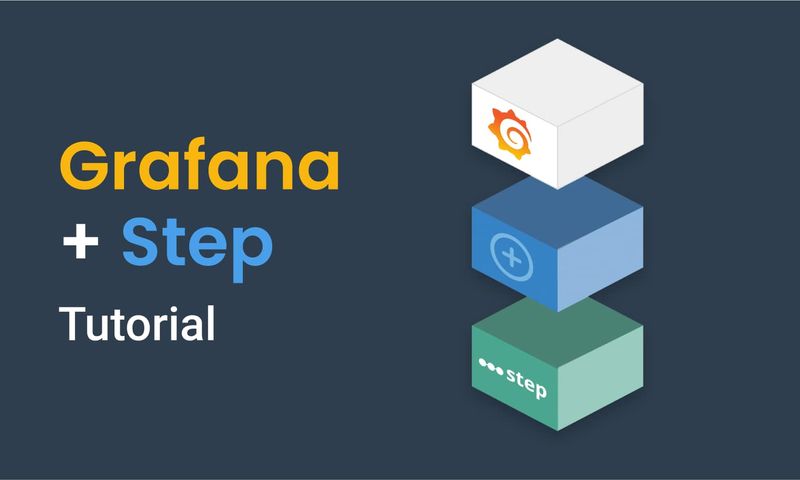
This article demonstrates how to connect Grafana to data generated by Step.
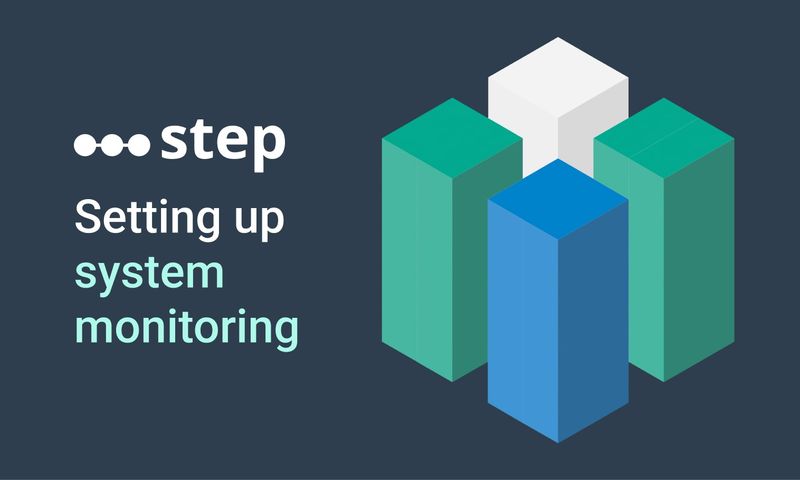
This article demonstrates how to set up distributed system monitoring using Keyword executions, and analyze the results as measurements.
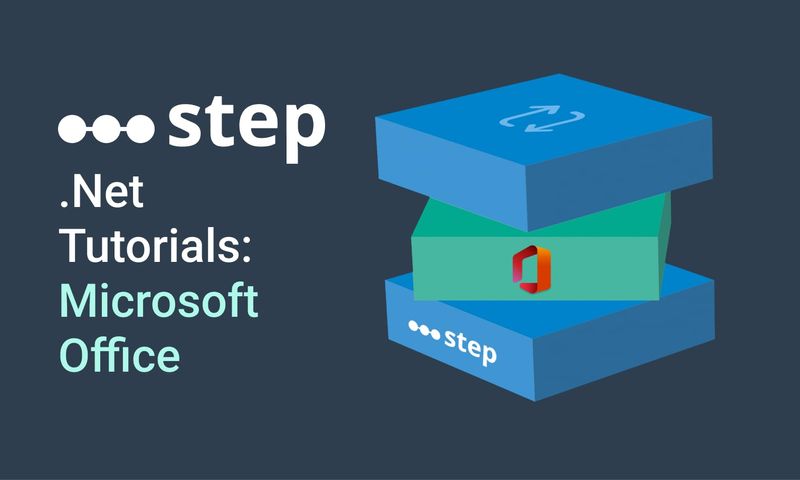
This tutorial demonstrates how to automate interaction with Microsoft Office applications using the Office Interop Assembly.
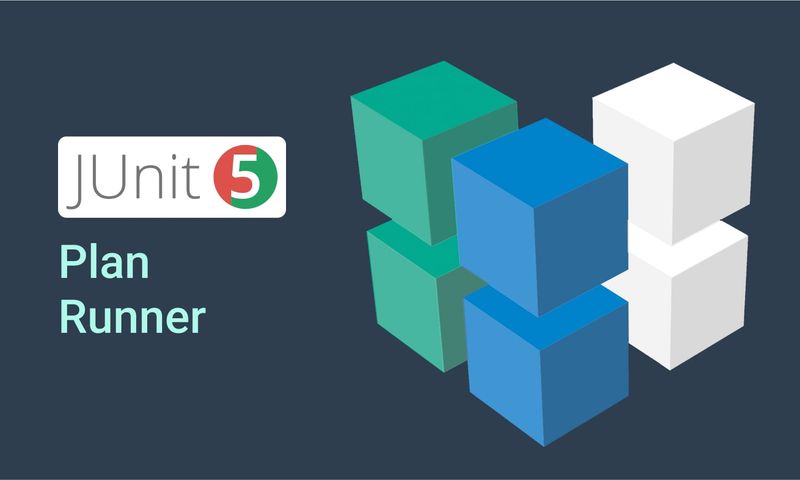
This article provides documentation for how to integrate JUnit tests into Step.
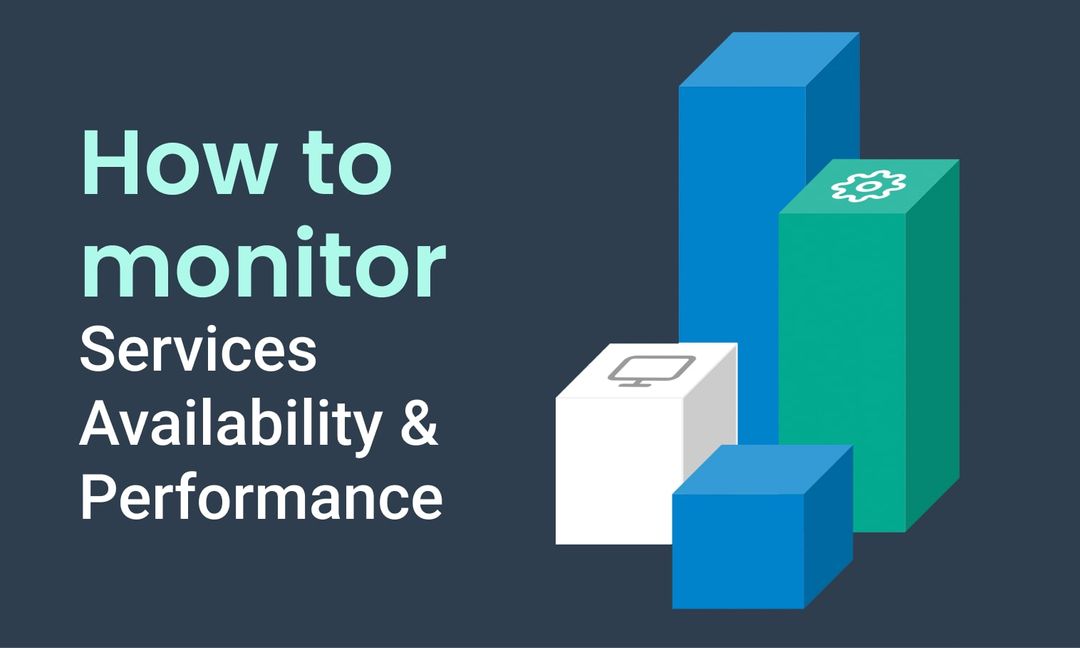
This tutorial demonstrates how Step can be used to monitor services, availability and performance metrics.
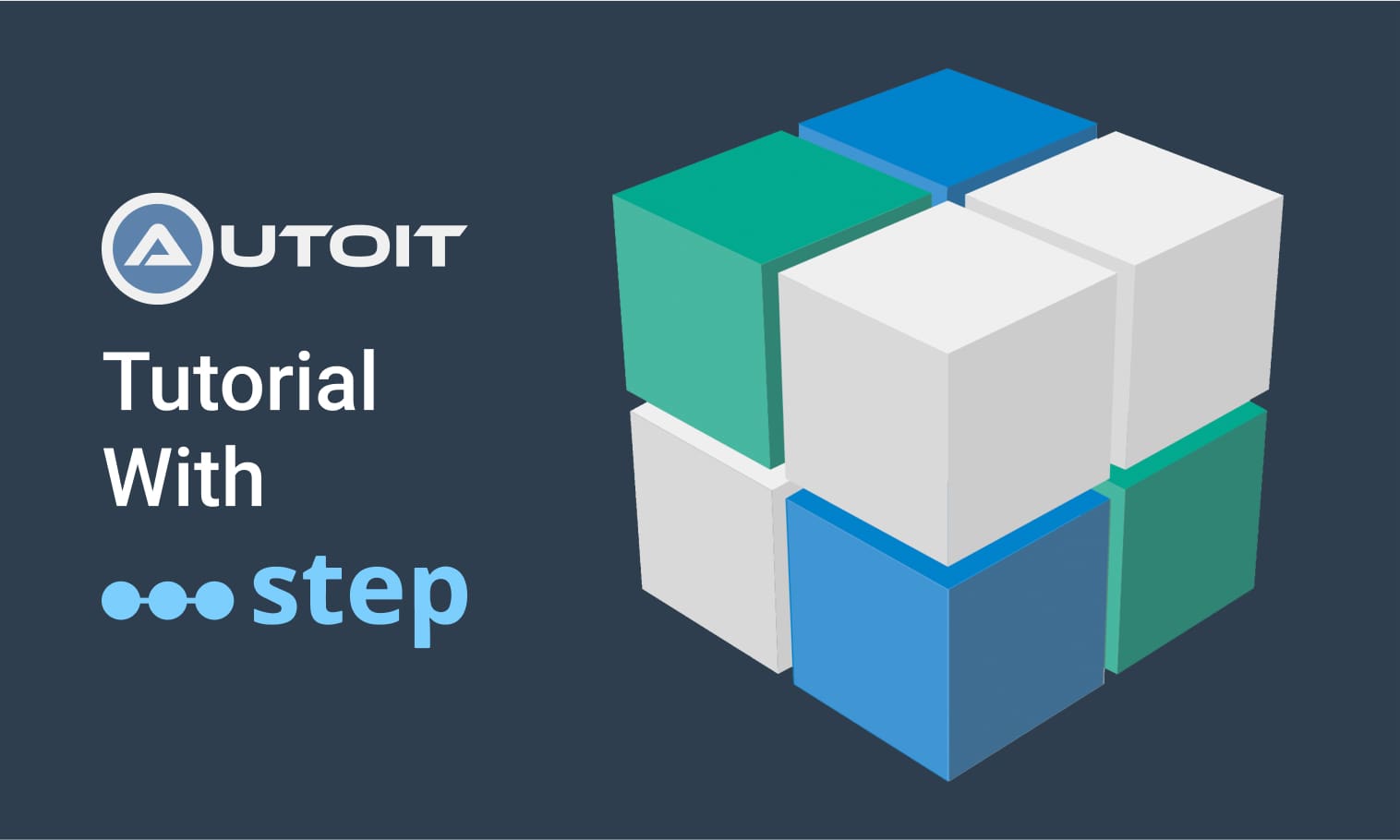
This tutorial demonstrates how to utilize the AutoIt C# binding to automate interactions with Windows applications.
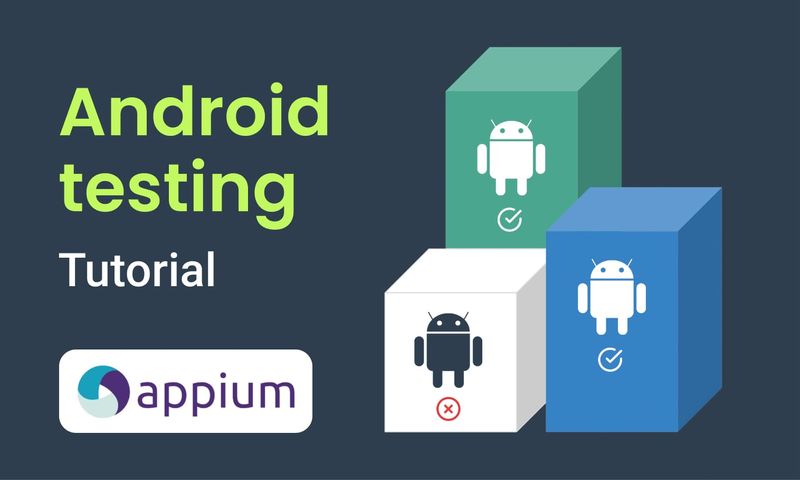
This article demonstrates the automation of mobile applications on Android using the Appium framework.
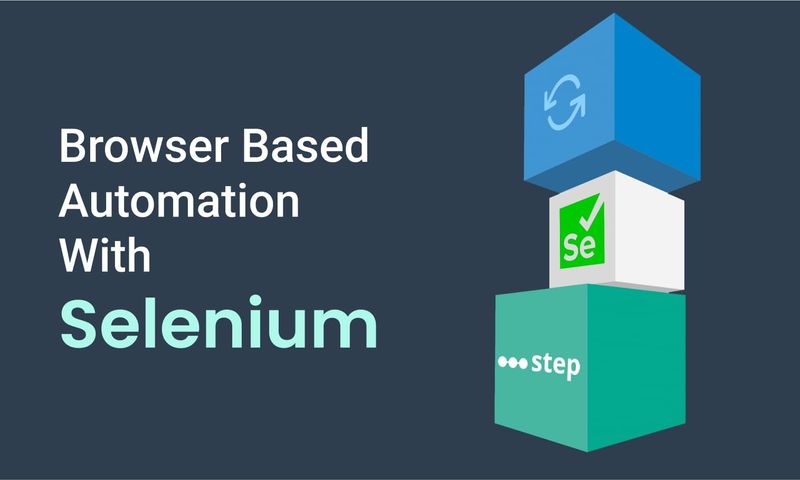
This article defines three Keywords which will be used in browser-based automation scenarios, using Step and Selenium, as general drivers.
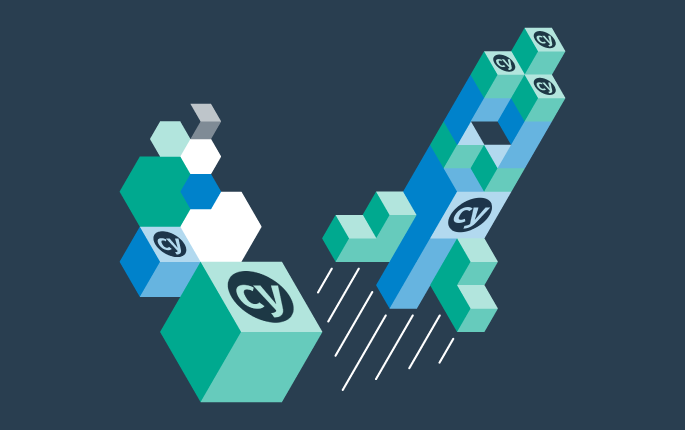
This tutorial shows you how to efficiently set up a browser-based load test using existing Cypress tests in the Step automation platform.
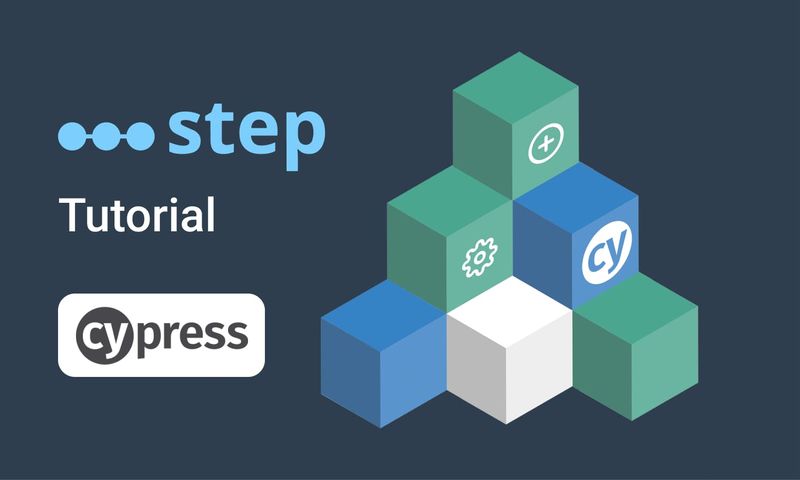
In this short tutorial, we show how to quickly implement a simple browser-based load test based on Cypress scripts in Step.
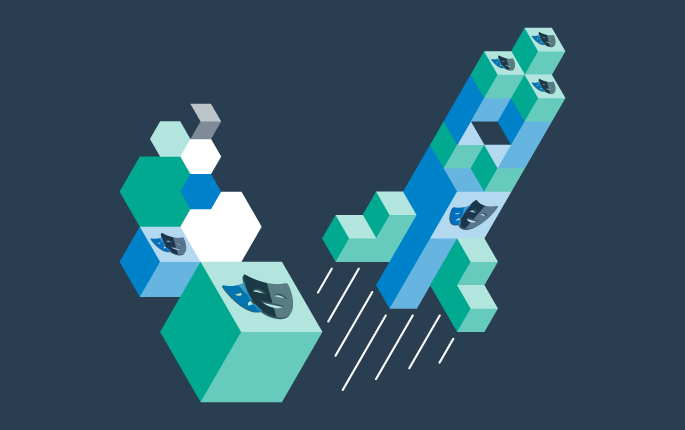
This tutorial shows you how to set up a browser-based load test using existing Playwright tests in the Step UI.
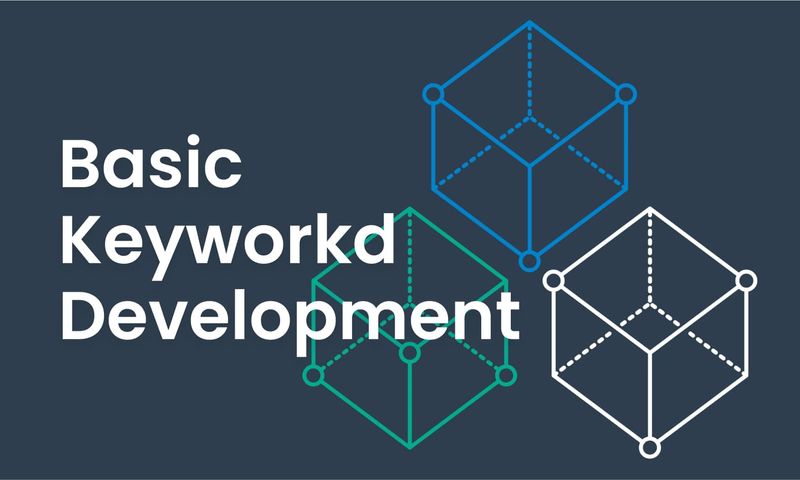
This article explains Keywords in Step and demonstrates how to create simple ones.
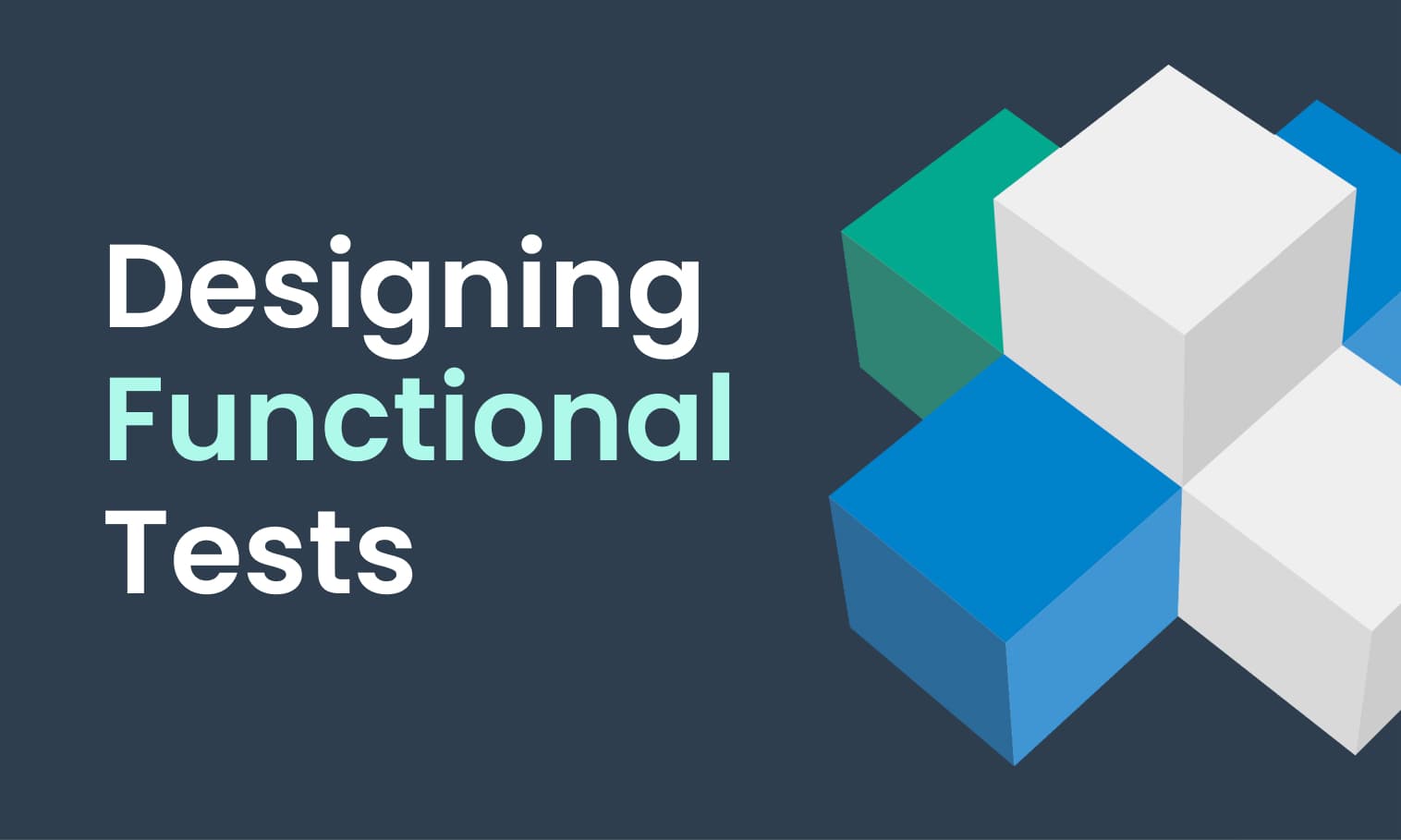
This tutorial demonstrates the design, execution, and analysis of functional tests using the web interface of Step.
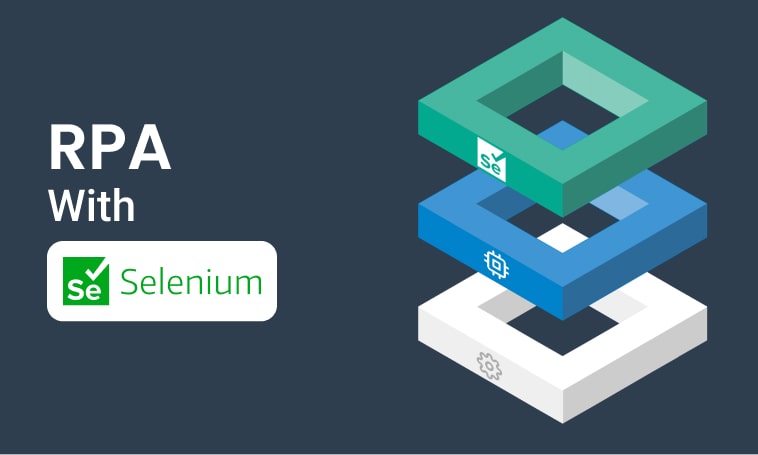
This tutorial will demonstrate how to use Step and Selenium to automate various browser tasks.
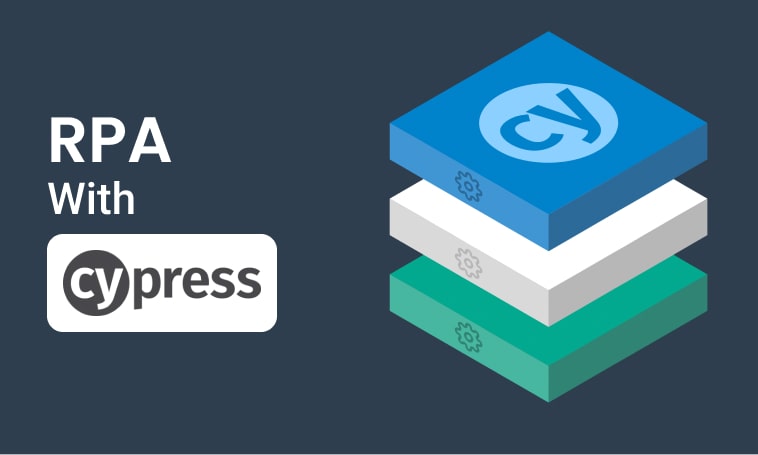
This tutorial demonstrates how to use Step and Cypress to automate various browser tasks.
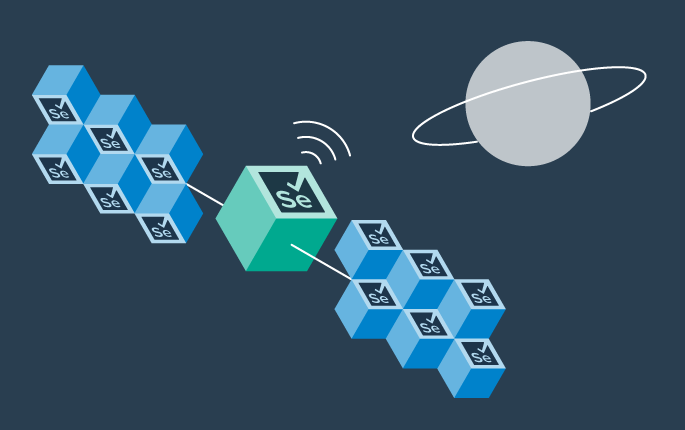
This tutorial demonstrates how Selenium automation tests can be turned into full synthetic monitoring using Step.
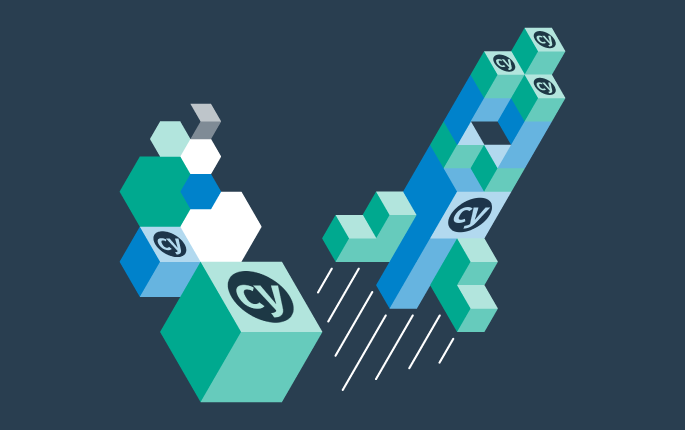
In this tutorial, you'll learn how to reuse existing Cypress tests to quickly set up and run a browser-based load test using the automation as code approach.
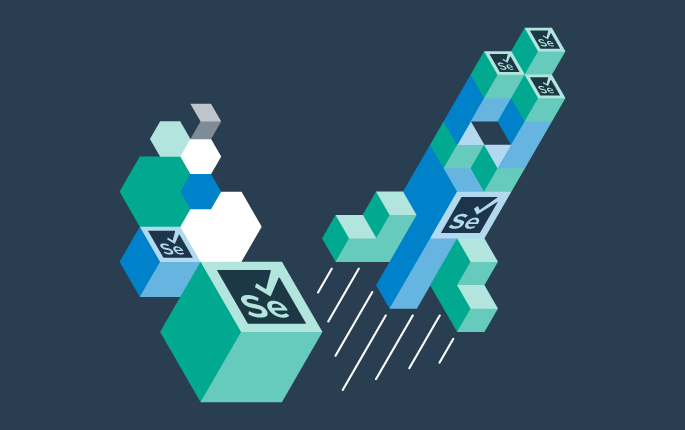
In this tutorial, you'll learn how to reuse existing tests written with Serenity BDD and Cucumber for load testing.
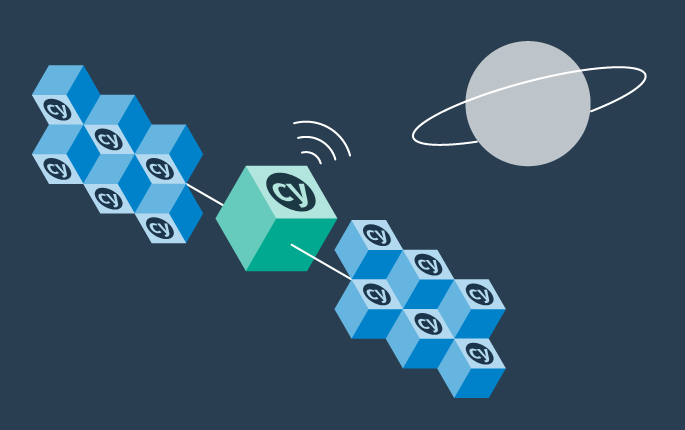
This tutorial demonstrates how Cypress automation tests can be turned into full synthetic monitoring using the automation as code approach.
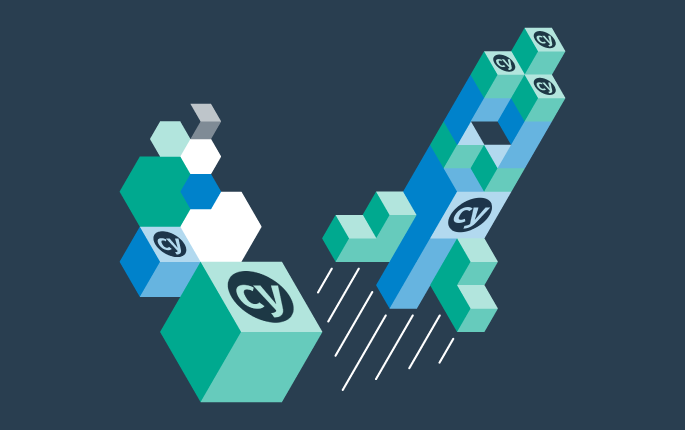
In this tutorial, you'll learn how to reuse existing Cypress tests to quickly set up and run a browser-based load test using the Step UI.
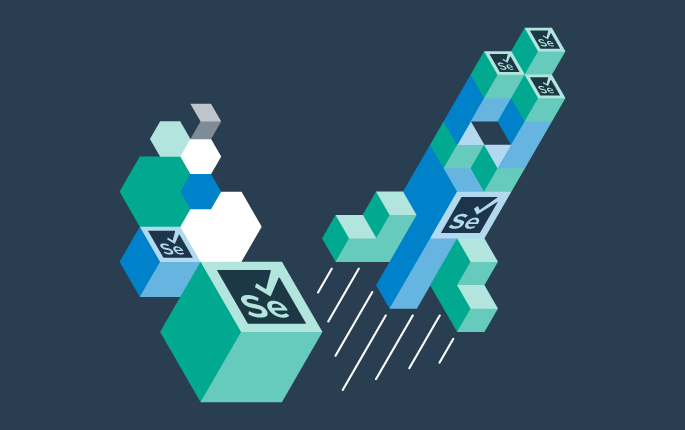
This tutorial demonstrates how to leverage existing Selenium tests to set up and execute browser-based load tests, following a full code-based approach.
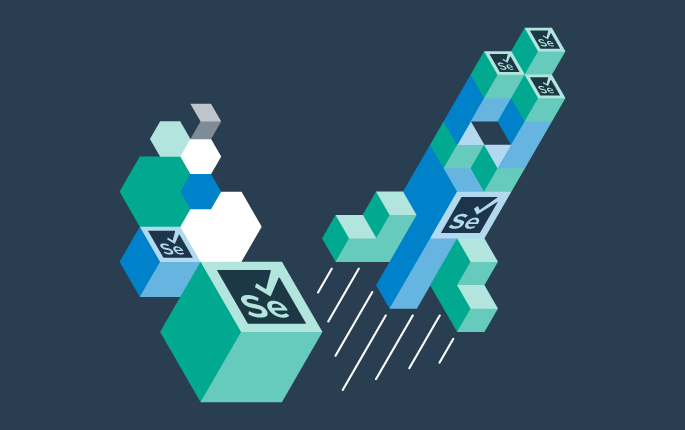
This tutorial demonstrates how to set up a browser-based load test in the Step UI using existing Selenium tests.
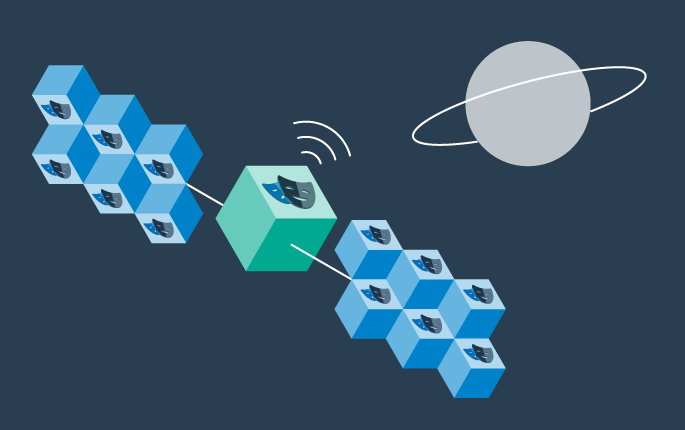
This tutorial demonstrates how Playwright automation tests can be turned into full synthetic monitoring using Step.
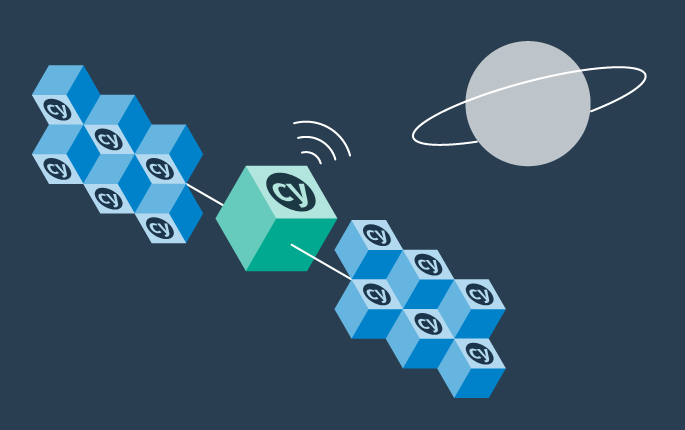
This tutorial demonstrates how Cypress automation tests can be turned into full synthetic monitoring using the Step UI.
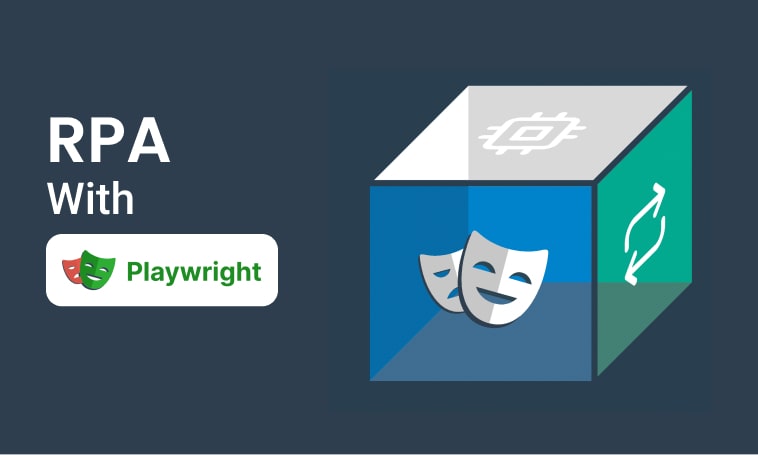
This tutorial will demonstrate how to use Step and Playwright to automate various browser tasks.
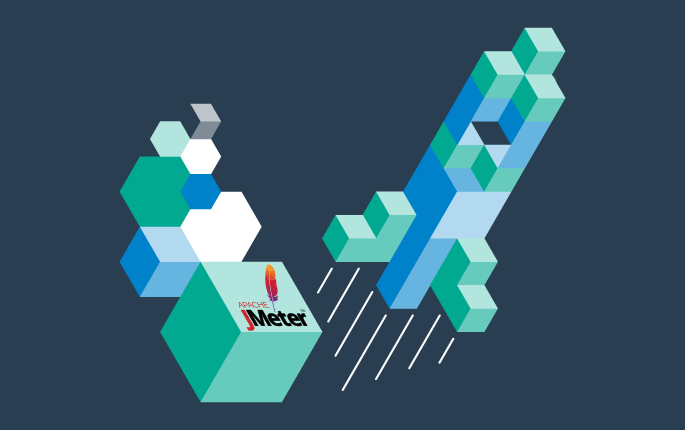
This tutorial shows how to distribute JMeter tests across multiple nodes.
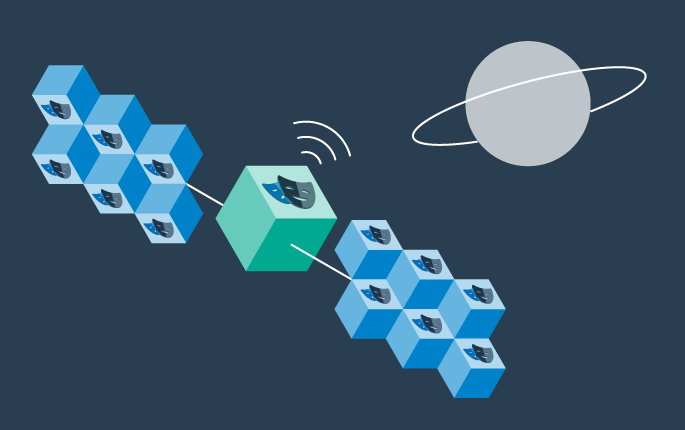
This tutorial demonstrates how Playwright tests can be reused for synthetic monitoring of a productive environment in a DevOps workflow
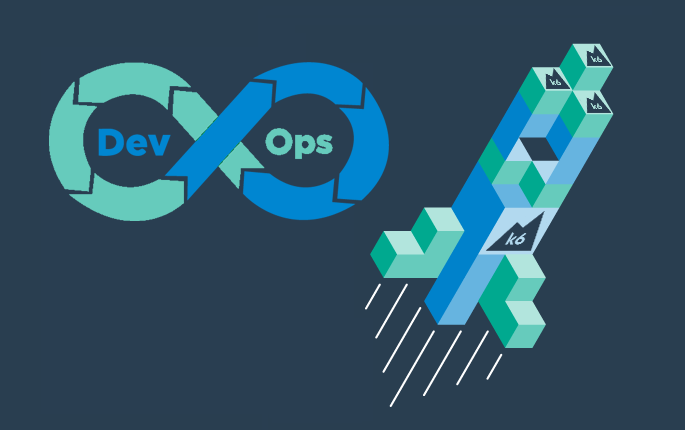
This tutorial shows how to distribute Grafana K6 tests across multiple nodes.
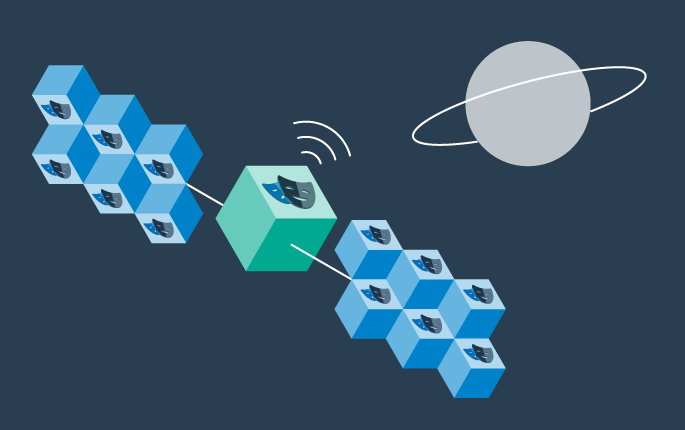
This tutorial demonstrates how Playwright tests can be reused for synthetic monitoring of a productive environment in a DevOps workflow
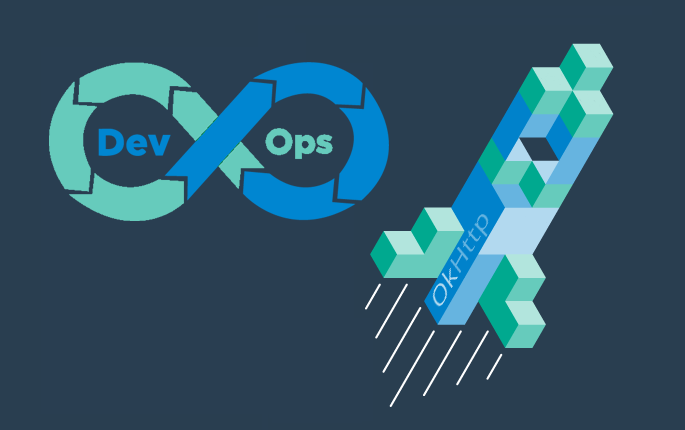
In this tutorial you'll learn how to quickly set up a protocol-based load test with okhttp
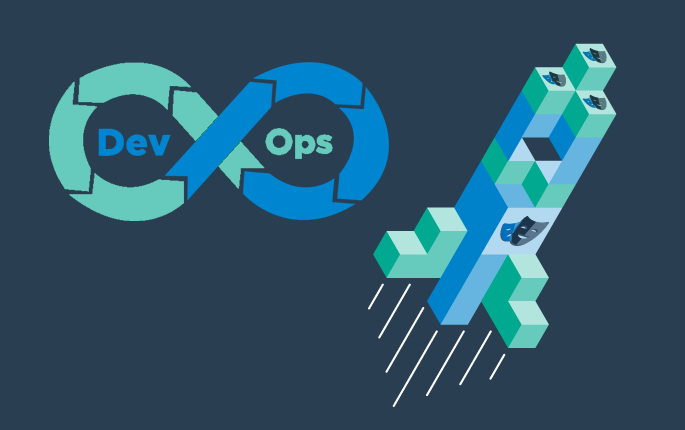
Learn how to set up continuous end-to-end testing across several applications based on Playwright tests in your DevOps pipeline using Step
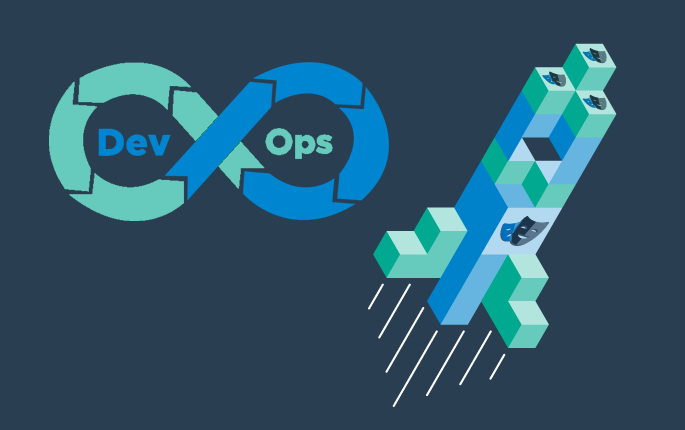
Learn how to quickly set up continuous browser-based load testing using Playwright tests in your DevOps pipeline
Want to hear our latest updates about automation?
Don't miss out on our regular blog posts - Subscribe now!