Keyword Development
Keywords are the fundamental building blocks of Plans. They encapsulate the automation logic and can represent fully automated flows or finer-grained actions, such as single user interactions or service calls.
While Step integrates with a wide range of automation tools and frameworks (like Cypress, K6, SoapUI, etc.) natively through plugin based keyword, it also supports custom keyword development for seamless integration with any others frameworks (Playwright, Selenium, Appium, ect.) thanks to the Step’s keyword API.
This page aims to highlight the concept and best practices that should be considered while developing your own custom keywords, refer to the keyword API page for the technical documentation.
What is a custom keyword
A custom keyword in Step is a user-defined block of automation logic implemented in code, extending the platform’s functionality to meet specific needs. Unlike predefined or plugin-based keywords, custom keywords allow you to write tailored routines in the supported programming language: Java, .NET, JavaScript, or TypeScript.
The Keyword API provides the tools needed to create custom keywords, it provides a clear interface to:
- Define and configure Keywords:
- Use annotations and class extensions to transform standard programming functions into Step-compatible keywords.
- Access Keyword inputs, session objects, and report data (keyword outputs, performance measurements, attachments)
- Implement hooks related to keyword executions (interceptors, error management)
Custom keywords empower developers to integrate any tool, framework, or custom logic into Step workflows, ensuring flexibility and scalability in automation.
Keyword Lifecycle
Key Points:
- The Keyword execution is stateless, in its simplest form it consists into instantiating and invoking the Keyword’s function, the keyword exists only for the time of its invocation
- The execution flow can be enriched by implementing the available and optional hooks
- For stateful executions, data can optionally be stored in the attached session.
The lifecycle of a keyword execution involves the following stages:
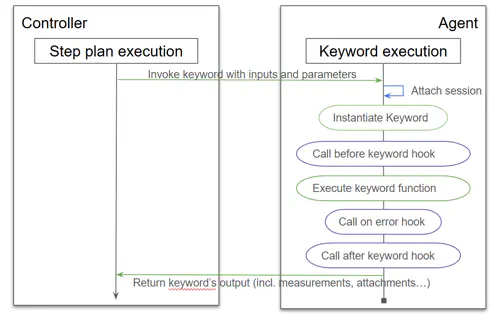
- Initialization:
- The keyword class is instantiated when the keyword is invoked, inputs and properties are set
- The session context is attached to the Keyword’s instance
- Execution flow:
- beforeKeyword Hook
optional
:- Called before the keyword’s main function is executed
- Use this to perform pre-execution setups (e.g., establishing connections or validating inputs)
- Keyword Function:
- The annotated method containing the logic for the keyword is executed
- onError Hook
optional
:- Triggered if an error occurs during the execution of the keyword
- Useful for error handling or rollback actions
- afterKeyword Hook
optional
:- Always called after the keyword function completes, regardless of success or failure
- Ideal for cleanup operations
- beforeKeyword Hook
- Report data: including keyword outputs, errors, performance measurements, attachments
- Instance Release:
- After execution, the keyword’s class instantiated in the initialization phase is released. As a result, ** keywords** do not retain any data between executions
When to use hooks
The simplest implementation of a keyword is to define only the keyword’s function. This allows you to include your automation code, resource cleanup, and error handling all within a single function.
Hooks, as described in the keyword lifecycle, can be used for more advanced scenarios. For instance, you might want to attach a screenshot in case of an error by using the onError hook.
Stateful Keywords Lifecycle
As mentioned above, keyword instances and executions is by definition stateless. However, the Step platform and its keyword API enable stateful executions of a group of keywords by sharing a session.
Key Points:
- Stateful keywords can store and retrieve data across executions using the session property
- The session is managed by the platform, allowing shared state across multiple keywords within the same workflow
- The session is only available for keywords calls which are grouped in a workflow using the Session control
The lifecycle is:
- The Session is initialized when the execution of the group starts
- The Session is a passed to each keyword invoked inside this group
- The session is closed right after the group of keywords is executed. All objects stored in the session by the keywords will be closed if and only if they implement “Closeable” or AutoCloseable" interfaces
Stateless vs. Stateful Keywords
While a keyword itself is stateless, the Step platform and keyword API allows stateful implementation by sharing a session across keywords’ executions.
Feature | Stateless Keywords | Stateful Keywords |
---|---|---|
Instance Lifecycle | New instance per execution. | Data shared via session property. |
Data Persistence | No persistence between executions. | Data persists across executions in the session. |
Use Case | Ideal for idempotent, isolated operations. | Useful for workflows requiring shared state. |
When to Use Stateful Keywords
- When a keyword needs to retain data for use in subsequent executions within the same workflow
- Example: Storing authentication tokens, counters, or temporary results
When to Use Stateless Keywords
- For lightweight, independent operations
- Example: Logging, stateless API calls, or simple data transformations
Best Practices
General Guidelines
- Minimize Dependencies:
- Avoid unnecessary dependencies to keep keywords lightweight
- Use Meaningful Names:
- Clearly name keywords to reflect their functionality
Keywords modularity
Whether your keywords encapsulates fully automated flows or finer-grained actions will impact the modularity, reusability but also the development effort of your keywords:
- Keywords performing a single, well-defined task increase its modularity and will enable to reuse the same automation code seamlessly for many different workflows and scenarios. The downside is higher development effort especially for re-using existing automation code
- On the other side larger keywords, wrapping entire workflow for example, will lose the modularity and reusability, but will require lower development effort
Resource Management
Resources include any object created by your code (or 3rd party libraries) requiring to be closed and cleanup explicitly including resources created directly on the filesystem. Commons examples are
- Closing browsers and web driver
- Releasing database connections
- Releasing file handles
- Generating or downloading documents on the filesystem
Clean Up resources approaches and best practices:
- Stateless keywords without hooks
- Ensure resources are closed within the keyword’s function
- Use language specific best practices such as try-with-resource construct when it applies
- Stateless keywords with custom hooks
- Use the
afterKeyword
hook to release resources
- Use the
- Stateful keywords
- For any data stored in a session that requires explicit cleanup, ensure it implements AutoCloseable or Closeable. As described in the stateful keywords lifecycle, the session is closed immediately after the group of keywords is executed, automatically closing all stored objects that support these interfaces.
Error Handling
Handle Errors Gracefully:
- Implement error handling in your code following language specific best practices
- Properly categorize errors with their message and type (business or technical error)
- Any uncaught exception will be reported as a technical error. It is advices to catch them and categorize them as describes above. This can be done directly in the keyword’s function or by using the
onError
hook.
Session Usage
- Use session properties sparingly to avoid memory overhead
- Objects stored in session must implement Closeable or AutoCloseable if they require to be cleaned-up once the session is released
FAQs
-
Can I use the same keyword instance for multiple executions? No, keyword instances are stateless by default. Use the session property for sharing data across executions.
-
What happens if error handling is not implemented? The platform will report back all exception as a technical error reusing the exception message and attaching its stack trace.
-
What happens if clean-up is not implemented? The platform will not perform automatic resource cleanup for resources created by the keyword code or by the used 3rd party libraries. Always implement resource management properly if your keyword creates temporary resources.
-
How can I debug my keywords? Keywords can be executed directly in your IDE with unit tests. For debugging executions on the platform you may use logging, the logs will be available in the logs of the agent that executed the keyword.
Additional resources
In addition to above documentation, you will find tutorials on the documentation pages as well as examples on github:
- Examples:
- Main classes in javadocs related to Keyword:
- Tutorials:
- Browse our tutorials provided for different automation framework and use cases