Event Broker API
EventBroker
The EventBroker Service and API allow asynchronous communication to happen in Step as well as synchronization between keywords, between plans and between keywords and plans. It is particularly useful in scenarios where a thread (or a “user”) needs to wait for specific events before performing the next task.
These events can be created internally (from within a keyword or plan) using various types of clients (Java, REST, prepopulated Keywords and Artefacts). The service also exposes methods for testing the presence of an event, reading it’s content, consuming it, etc.
Id’s vs Groups
Events can be declared and targeted either through a unique identifier or a group & name combination. This is an important choice as it will impact the way you’ll be using the API and how Events are managed. Here’s the underlying reason behind each of these approaches:
Id-based declaration
Setting a unique identifier in the Id field of the Event object, as you could assume, will allow for the explicit manipulation of that event instance and only that event instance. This could be seen as the standard scenario in which the consumer of the event knows exactly what event they are waiting for and how to identify it.
It is important to note that the consumer has to be aware of the **exact **identifier ahead of time, or it will not be able to retrieve the event. Since the identifier is unique and is set dynamically, this is a strong constraint.
Group & Name declaration
Groups are designed to gather and store distinct, yet similar events. You can see this as a relaxed constraint on the identifier as consumers will be able to consumer any event from the group and no guarantee will be provided as to which Event instance specifically will be resolved. In other words, groups allow for the management of pools of events which share a common purpose.
Consumers only need to be aware of the Group identifier, which is a static string. In addition to the Group field, providers have the option to also set a name for the Event. The name field provides consumers with the ability to uniquely identify an event after it’s been retrieved based it’s Group.
Using the Java API
The STEPClient class provides access to a RemoteEventBrokerClient class, which exposes most of the broker’s primitives.
Primitives
- Put:upload an event to the broker
- Peek: download a copy of the event, leaving the existing event untouched in the Broker
- Consume: download and exhaust the event
Example
Initializing the client:
RemoteEventBrokerClient client = new StepClient("http://my-step-controller:8080", "admin", "init").getEventBrokerClient();
Event sentEvent = new Event().setId("a_unique_id").setPayload(new Integer(10));
client.putEvent(sentEvent);
client.peekEvent("a_unique_id");
Event readEvent = client.consumeEvent("a_unique_id");
System.out.println(readEvent);
Event sentEvent = new Event().setGroup("myGroup").setName("myEventName").setPayload(new Boolean(true));
client.putEvent(sentEvent);
client.peekEventByGroupAndName("myGroup", "myEventName");
Event readEvent = client.consumeEventByGroupAndName("myGroup", "myEventName");
System.out.println(readEvent);
client.close();
Artefacts and Keywords
Various artefacts and keywords have been pre-populated in Step for convenience.
WaitForEvent artefact
Assuming some event of group “myGroup” and of name “myEventName"is sent by a third party EventBrokerClient, the following plan will wait for the event to be received and then store the content of the event in the variable “result”:
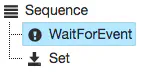
**WaitForEvent **configuration:
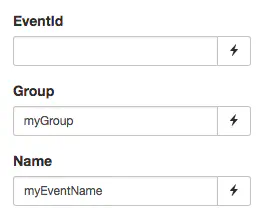
Set configuration:
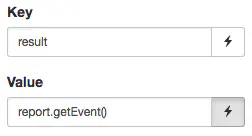
The same applies with the Id-based approach, i.e you just need to leave the Group & Name fields empty and only set the Id.
Put, Peek & Get keywords
LocalFunction keywords have been pre-populated in Step. This means they are available upon starting the controller for the first time. LocalFunction’s are a special type of keyword which is executed directly in the controller. However, these keywords can also be manually deployed as regular Java keywords.
Schemas are also pre-configured for convenience. This means that the inputs can easily be added by selecting “Add input” and then clicking on the desired fields key:
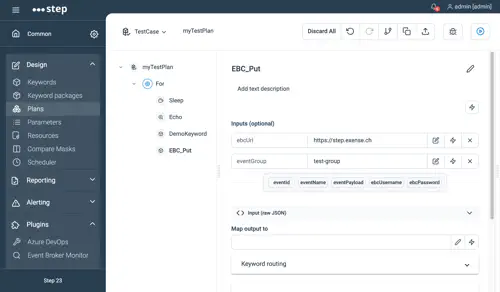
These keywords can either target the local controller (for example, using “http://localhost:8080” as the ebcUrl) or a remote controller.
Put, Peek and Get respectively match the Put, Peek and Consume primitives with the difference that for the Put, the payload field (called “payloadAsJson”) needs to be fullfilled with a valid Json string.
EventBrokerMonitor
A view is available to monitor your events as described here